Houston, we have a 404.
We can't seem to find the page you were looking for. Either the URL was mistyped, never existed, or once existed but was mysteriously lost.
Here are some helpful links instead:
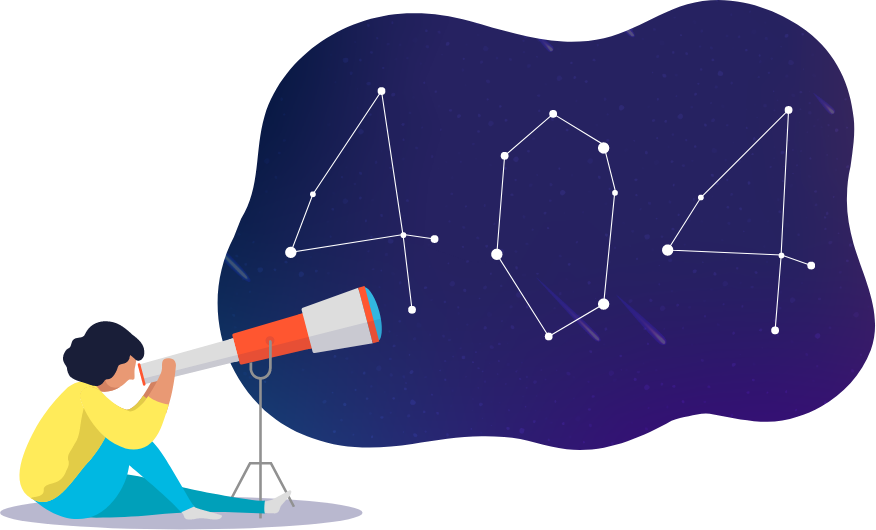
We can't seem to find the page you were looking for. Either the URL was mistyped, never existed, or once existed but was mysteriously lost.
Here are some helpful links instead: